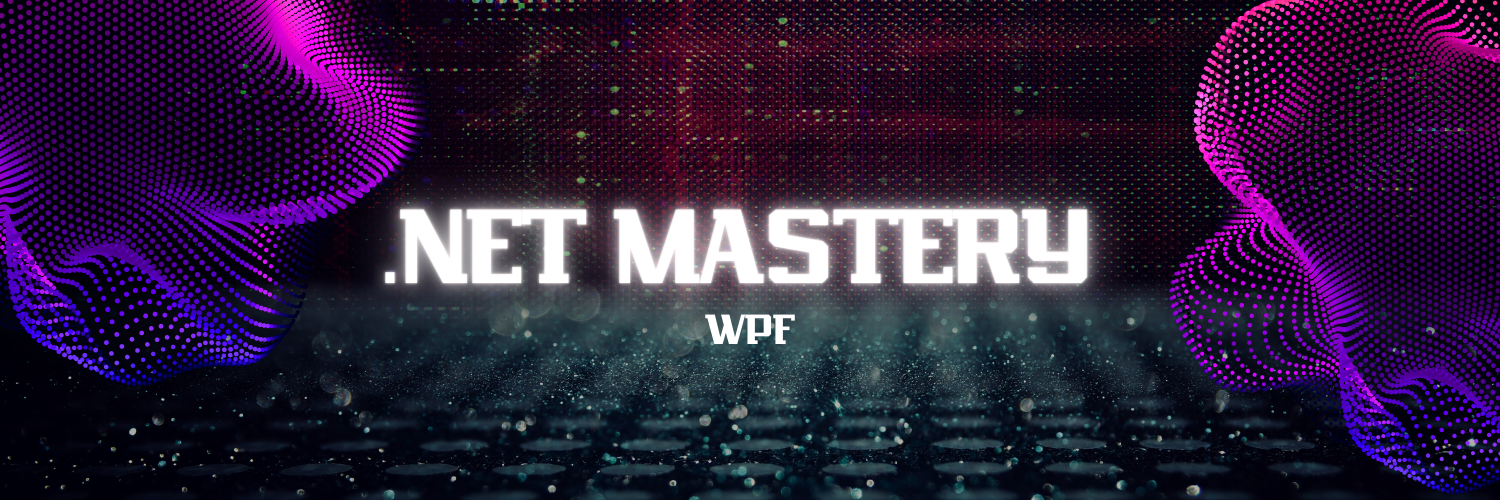
App.xaml - One file to rule them all
App.xaml - One file to rule them all
Intro
App.xaml is your application main file and is automatically created. It defines what your app will do when started, suspended, onbackground, etc.
When created, the file look something like this
<Application
x:Class="MySolution.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
StartupUri="MainWindow.xaml">
<Application.Resources>
</Application.Resources>
</Application>
Let’s explore the main features that we can use in this file.
Properties
StartupUri
The most important property of an application is StartupUri, this prop specifies the UI that automatically opens when an application starts. So, if you do
<Application
x:Class="MySolution.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
StartupUri="Biscoito.xaml">
<Application.Resources>
</Application.Resources>
</Application>
Biscoito.xaml will open when you run the app.
You can see the full list of suported UIs that can be set on StartupUri here.
Resources
With this property we can set a collection of application-scope resources, such as styles, brushes and converters. It’s great to create a general theme for the app, being thread safe and available from any thread.
We can set a Resource from code-behind
Application.Current.Resources["ApplicationScopeResource"] = Brushes.White;
Or throuth the .xaml
<Application
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="MyApp.App"
StartupUri="MainWindow.xaml">
<Application.Resources>
<SolidColorBrush x:Key="MyWhite" Color="White"></SolidColorBrush>
</Application.Resources>
</Application>
either way the solid brush will be available for all the assembly.
We can also get the resource via code-behind
Brush whiteBrush = (Brush)Application.Current.Resources["MyWhite"];
or .xaml
<TextBlock Text="Biscoito" Foreground="{StaticResource MyWhite}"/>
Resources are a good way to implement a theme for the app, ‘cause if resources change, the resource system ensures that elements properties which are bounded to those resources are automatically updated to reflect changes.
Events
One of the coolest things on c# are events, and when talking about the Application class we cannot go on without talking about some of them. Here we will see the 3 most interesting ones:
- Startup;
- DispatcherUnhandledException;
- Exit.
Startup
This event is called when the Run() method is invoked; therefore, after the application execution model has been established.
Startup can be of great value when dealing with parameters or if we want to define some properties and/or datacontext of our main window before it is initialized.
The documentation has one good example illustrating parameters handling.
DispatcherUnhandledException
This event is called whenever an exception is throw by the main UI thread. So subscribing to it let we handle the exceptions we are not expected to happen (not used a try/catch)
It’s important to notice that if the exceptions are throw by a background UI thread or a background worker it will never get through the main UI thread.
Therefore, once we subscribe to the event.
<Application
x:Class="WpfTutorialSamples.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
DispatcherUnhandledException="Application_DispatcherUnhandledException"
StartupUri="MainWindow.xaml">
<Application.Resources>
</Application.Resources>
</Application>
We just need to implement how we want to handle the exception.
namespace MySolution
{
public partial class App : Application
{
private void Application_DispatcherUnhandledException(object sender,
DispatcherUnhandledExceptionEventArgs e)
{
MessageBox.Show(
$"An unhandled exception just occurred: {e.Exception.Message}",
"Exception Sample",
MessageBoxButton.OK,
MessageBoxImage.Warning);
e.Handled = true;
}
}
}
You could ask why we set `e.Handled = true` and would be a good question! If you look at the documentation you will read that when a unhandled exceptions throw the Windows Run Time will close the app unless that the exception is setted as handle.
Be aware that some times we have no choice but let the WRT kill the application. We can decide that looking in the parameter; e.Exception will say if the exception was just a naive FileNotFoundException or an unrecovable StackOverflowException.
Exit
Occurs just before an application shuts down, and cannot be canceled. It’s useful when we want to do different things depending on the reason the app was closed.
For example, if the application is closing because of an exeption, we can send an email with the crash logs or save the state of the app and, overriding the OnLaunched, recover the state once the app is restarted.
Documentation
You should also read the full documentation.